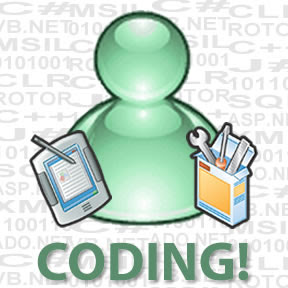
I happened to read a book called "Software Development Guidelines", I will list out the points from this book which I liked.
- Let each routine designed perform an unique task. Divide the program into routines such that each of them performs a separate task. Let all routines be clearly distinguishable.
- Do not make any routine too long. Also do not simply divide the routines without any logical need. If a routine is more than 100 lines one may try to divide it if it can be divided into routines which can perform separate tasks.
- Let the routines be loosely coupled. (pass less parameters, make the routine interfaces flexible, make them more visible )
- Let all the files related to a module be in one directory.
- Let all modules be divided logically. The interfaces should be provided for interaction between modules
- All other internal data within module should be private
- Declare int, float and all as typedef e.g typedef int int16 (on 16 bit machine) so that one need not go and the variable type everywhere. This makes the program more platforms independent.
- Do explicit declarations of all variables before it is used.
- Declare all the variables , constants at the beginning of the routine.
- Every variable declared should have a comment describing the need, function and limitation of the variable.
- Better to declare each variable in a separate line with its description
- Always use meaningful names for the variables , this increases the readability of the program like anything (have high quality identifiers )
- Do not create two different variables in the program which only differ in their cases (upper case or lower case) because not all compilers are case sensitive. To make program portable use case neutral variables.
- Capitalize the first letter of interior words in all multi-word identifiers.
- Variable names should be meaningful, concise, and non-ambiguous to an average programmer fluent in the English language.
- Try to avoid abbreviated variable names .If used give description of the abbreviated variable names.
- Never use a numeric suffix to differentiate two names.
- Try to make most identifiers unique in the first few character positions of the identifier. This makes the program easier to read.
- If your code contains a chain of if..elseif..elseif.......elseif..... statements, do not use the final else clause to handle a remaining case. Only use the final else to catch an error condition. If you need to test for some value in an if..elseif..elseif.... chain, always test the value in an if or elseif statement.
- Always use the most appropriate type of loop (categorized by termination test position). Never force one type of loop to behave like another.
- Avoid empty loops. If testing the loop termination condition produces some side effect that is the whole purpose of the loop, move that side effect into the body of the loop. If a loop truly has an empty body, place a comment like "/* nothing */" or "{null statement}" within your code.
- Loops with a single exit point are more easily understood
- All loops should have one entry point. The program should enter the loop with the instruction at the top of the loop.
- Make each loop perform only one function.
- Code, as much as possible, should read from top to bottom( do not use lot of goto statements , deeply nested if else with lot of possibility of code jumping to some other location )
- An expression should not produce any side effects.
- Do not user lesser used operators, porting to different language may be difficult.
- Use parentheses where ever necessary. We can do without parentheses for mul, division, addition, and subtraction but for others different languages, different compliers may have different precedence hence better user brackets.
- Indentation should be three to four spaces in an indented control structure with four spaces probably being the optimal value.
- If you use tabs to indent your code, insert a comment at the very beginning of the program that states the number of positions for each tab stop. E.g., "/* this program is formatted using four character position tab stops. */"
- All related statements should be together, there should not be blank lines in between them.
- Blank lines should be present in between of declaration and start of code.
- Blank should be present in between comment block and code
- Whenever there is different logical block blank line should be present.
- Source code lines will not exceed 80 characters in length
- Always put a blank line between any block statement and the statement(s) it encloses
- Write comments that describe blocks of statements rather than individual statements. Comments covering single statements tend to discuss the mechanics of that statement rather than discussing what the program is doing.
- Focus paragraph comments on the why rather than the how. Code should explain what the program is doing and why the programmer chose to do it that way rather than explain what each individual statement is doing.
- Use comments to prepare the reader for what is to follow. Someone reading the comments should be able to have a good idea of what the following code does without actually looking at the code. Note that this rule also suggests that comments should always precede the code to which they apply.
- All comments will be high-quality comments that describe the actions of the surrounding code in a concise manner.
- All comments will be up to date. If a programmer makes changes to the code, that programmer is responsible for updating the internal comments and any external documentation affected by those changes.
- All inter module communication in C/C++ programs must take place through header files (“.h” files). All extern directives, public class definitions, public type definitions, and public constants must appear in the header file that all interested modules will include.
- For functions like malloc which is used at many places we can used a customized safe_malloc for our C application so that the necessary check is also included in the safe_malloc function.